How does instagram load images so fast
Making Instagram.com faster: Part 1 | by Glenn Conner
In recent years, instagram.com has seen a lot of changes — we’ve launched stories, filters, creation tools, notifications, and direct messaging as well as myriad other features and enhancements. However, as the product grew, one unfortunate side effect was that our web performance began to suffer. Over the last year, we made a conscious effort to improve this. Our ongoing efforts have thus far resulted in almost 50% cumulative improvement to our feed page load time. This series of blog posts will outline some of the work we’ve done that led to these improvements.
Performance improvements over the last year for feed page Display Done times (milliseconds)Correctly prioritizing resource download and execution and reducing browser downtime during the page load is one of the main levers for improving web application performance. In our case, many of these types of optimizations proved to be more immediately impactful than code size reductions, which tended to be individually small and only began to add up after many incremental improvements (though we will talk about these in future installments). They were also less disruptive to product development, requiring less code change and refactoring. So initially, we focused our efforts in this area, beginning with resource prefetching.
JavaScript, XHR, and image prefetching (and how you need to be careful)
As a general principle, we want to inform the browser as early as possible about what resources are required to load the page. As developers, we often know what resources we are going to need ahead of time, but the browser may not become aware of those until late in the page loading process. These resources mainly include those that are dynamically fetched by JavaScript (other scripts, images, XHR requests etc.) since the browser is unable to discover these dependent resources until it has parsed and executed some other JavaScript first.
Instead of waiting for the browser to discover these resources itself, we can provide a hint to the browser that it should start working on fetching those resources immediately. The way we do this is by using HTML preload tags. They look something like this:
<link rel="preload" href="my-js-file.js" as="script" type="text/javascript" />
At Instagram, we use these preload hints for two types of resources on the critical page loading path: dynamically loaded JavaScript and preloading XHR GraphQL requests for data. Dynamically loaded scripts are those that are loaded via import('...')
for a particular client-side route. We maintain a list of mappings between server-side entrypoints and client-side route scripts — so when we receive a page request on the server-side, we know which client-side route scripts will be required for a particular server-side entrypoint and we can add a preload for these route specific scripts as we render the initial page HTML.
For example, for the FeedPage entrypoint, we know that our client-side router will eventually make a request for FeedPageContainer.js, so we can add the following:
<link rel="preload" href="/static/FeedPageContainer.js" as="script" type="text/javascript" />
Similarly, if we know that a particular GraphQL request is going to be made for a particular page entrypoint, then we should preload that XHR request. This is particularly important as these GraphQL queries can sometimes take a long time and the page can't render until this data is available. Because of this, we want to get the server working on generating the response as early as possible in the page lifecycle.
<link rel="preload" href="/graphql/query?id=12345" as="fetch" type="application/json" />
The changes to the page load behavior are more obvious on slower connections. With a simulated fast 3G connection (the first waterfall below -without any preloading), we see that FeedPageContainer.js and its associated GraphQL query only begin once Consumer.js has finished loading. However, in the case of preloading, both FeedPageContainer.js and its GraphQL query can begin loading as soon as the page HTML is available. This also reduces the time to load any non-critical lazy loaded scripts, which can be seen in the second waterfall. Here FeedSidebarContainer.js and ActivityFeedBox.js (which depend upon FeedPageContainer.js) begin loading almost immediately after Consumer.js has completed.
Benefits of preload prioritization
In addition to starting the download of resources sooner, link preloads also have the additional benefit of increasing the network priority of async script downloads. This becomes important for async scripts on the critical loading path because the default priority for these is Low. This means that XHR requests and images in the viewport will have higher network priority, and images outside the viewport will have the same network priority. This can cause situations where critical scripts required for rendering the page are blocked or have to share bandwidth with other resources (if you’re interested, see here for an in-depth discussion of resource priorities in Chrome). Careful use (more on that in a minute) of preloads gives an important level of control over how we want the browser to prioritize content during initial loads in cases where we know which resources should be prioritized.
Problems with preload prioritization
The problem with preloads is that with the extra control it provides, comes the extra responsibility of correctly setting the resource priorities. For example, when testing in regions with very slow overall mobile and wifi networks and significant packet loss, we noticed that <link rel="preload" as="script">
network requests for scripts were being prioritized over the <script />
tags of the JavaScript bundles on the critical page rendering path, resulting in an increase in overall page load time.
This stemmed from how we were laying out the preload tags on our pages. We were only putting preload hints for bundles that were going to be downloaded asynchronously as part of the current page by the client-side router.
In the example for the logged out page, we were prematurely downloading (preloading) SomeConsumerRoute.js before Common.js & Consumer.js and since preloaded resources are downloaded with the highest priority but are not parsed/compiled, they blocked Common & Consumer from being able to start parsing/compiling. The Chrome Data saver team also found similar issues with preloads and wrote about their solution here. In their case, they opted to always put preloads for async resources after the script tag of the resource that requests them. In our case we opted for a slightly different approach. We decided to have a preload tag for all script resources and to place them in the order that they would be needed. This ensured that we were able to start preloading all script resources as early as possible in the page (including synchronous script tags that couldn’t be rendered into the HTML until after certain server side data was added to the page), and ensured that we could control the ordering of script resource loading.
Image prefetching
One of the main surfaces on instagram.com is the Feed, consisting of an infinite scrolling feed of images and videos. We implement this by loading an initial batch of posts and then loading additional batches as the user scrolls down the feed. However, we don’t want the user to wait every time they get to the bottom of the feed (while we load a new batch of posts), so it’s very important for the user experience that we load in new batches before the user hits the end of their current feed.
This is quite tricky to do in practice for a few reasons:
- We don’t want off-screen batch loading to take CPU and bandwidth priority away from parts of the feed the user is currently viewing.
- We don’t want to waste user bandwidth by being over-eager with preloading posts the user might not ever bother scrolling down to see, but on the other hand if we don’t preload enough, the user will frequently hit the end of feed.
- Instagram.com is designed to work on a variety of screen sizes and devices, so we display feed images using the img
srcset
attribute (which lets the browser decide which image resolution to use based on the users screen size). This means it's not easy to determine which image resolution we should preload & risks preloading images the browser will never use.
The approach we used to solve the problem was to build a prioritized task abstraction that handles queueing of asynchronous work (in this case, a prefetch for the next batch of feed posts). This prefetch task is initially queued at an idle priority (using requestIdleCallback), so it won’t begin unless the browser is not doing any other important work. However if the user scrolls close enough to the end of the current feed, we increase the priority of this prefetch task to ‘high’ by cancelling the pending idle callback and thus firing off the prefetch immediately.
Once the JSON data for the next batch of posts arrives, we queue a sequential background prefetch of all the images in that preloaded batch of posts. We prefetch these sequentially in the order the posts are displayed in the feed rather than in parallel, so that we can prioritize the download and display of images in posts closest to the user’s viewport. In order to ensure we actually prefetch the correct size of the image, we use a hidden media prefetch component whose dimensions match the current feed. Inside this component is an
<img>
that uses a srcset
attribute, the same as for a real feed post. This means that we can leave the selection of the image to prefetch up to the browser, ensuring it will use the same logic for selecting the correct image to display on the media prefetch component as it does when rendering the real feed post. It also means that we can prefetch images on other surfaces on the site — such as profile pages — as long as we use a media prefetch component set to the same dimensions as the target display component.
The combined effect of this was a 25% reduction in time taken to load photos (i. e. the time between a feed post being added to the DOM and the image in that post actually loading and becoming visible) as well as a 56% reduction in the amount of time users spent waiting at the end of their feed for the next page.
Stay tuned for part 2
Stay tuned for part 2: Early flushing and progressive HTML (and why you don’t necessarily need HTTP/2 push for pushing resources to the browser). If you want to learn more about this work or are interested in joining one of our engineering teams, please visit our careers page, follow us on Facebook or on Twitter.
Send Fast, Match Data Later
Story by
Matt Brian
Story by
Matt Brian
Matt is the former News Editor for The Next Web. You can follow him on Twitter, subscribe to his updates on Facebook and catch up with him (show all) Matt is the former News Editor for The Next Web. You can follow him on Twitter, subscribe to his updates on Facebook and catch up with him on Google+.
Smartphone photo-sharing app Instagram has struck a chord with users, making it easy to snap, modify and share their images, but one of its most impressive features is the ability to upload those photos in a super-quick fashion.
Instagram might now be part of the Facebook stable, and about to see input from staff employed for the world’s largest social network, but the app’s original creators Mike Krieger and Kevin Systrom knew that one of the keys to success was to make its “mobile experiences feel lightning-fast,” filling in gaps whilst their users worked within the app.
In a presentation at Warm Gun 2011 last December, Krieger detailed how Instagram’s iOS app was designed to process any form of input as it is entered, “moving bits in the background” as the user interacts with the app’s various features.
Get your tickets for TNW Valencia in March!
The heart of tech is coming to the heart of the Mediterranean
Join now
It’s the same for Instagram’s image uploads:
What Instagram does is to upload the image as soon as the user selects the filter and hits the green tick, entering the sharing screen — regardless of if you post it or not. The app makes “two requests, two round-trips”, sending the data as soon as part of it is ready to go, matching up the rest of the data later.
Instagram’s Krieger stated in his presentation in December that it is “worth it, even if you throw out the data on cancel.”
What you see as the user is a super-quick upload as soon as you hit ‘Done’, but behind the scenes the app works to process your data, as you enter it. It’s just one of the small things that helped the app reach 50 million users on just two platforms, leading to a $1 billion acquisition by Facebook.
See also: Facebook promised Instagram $200 million if the acquisition gets whacked by the government
How to add photos to Instagram from a computer in 2021 - detailed instructions This is done using:
- available browser tools (using four programs as an example);
- extensions for Chrome (on the example of two);
- deferred posting service;
- Creator Studio via Facebook*;
- Instagram promotion service*; nine0008
- Android and iOS emulator on PC;
- online services;
- applications from the Microsoft website (on the example of two).
Below we will analyze each of them.
With the help of available browser tools
This method is suitable for those who want to download infrequently and little.
How to add a photo to Instagram* via Google Chrome
To start uploading a photo:
- Log in to Instagram* via Google Chrome or its extended version of Cent Browser on your computer. nine0008
- Press F12 (or Shift+Ctrl+I, or right-click anywhere on the page, then View Code).
- In the developer window that appears on the right, click the phone icon.
- Refresh the page.
- Use.
How to upload a photo to Instagram* using Mozilla Firefox
- Access your Instagram page* through Mozilla Firefox on your computer.
- Press F12 (or Shift+Ctrl+I, or right-click anywhere on the page, then "Inspect Element").
- In the developer window that appears, click the phone icon.
- On the Responsive Design Mode line that appears, select any gadget model.
nine0008
- Refresh the page.
- Done! Post your new photos on Instagram*.
- If you don't like the developer window at the bottom of the page, move it on the screen to the right.
How to upload a photo to Instagram* via Opera
To post a photo:
- Access your Instagram profile* via the Opera browser on your computer.
- Press F12 (or Shift+Ctrl+I, or right-click anywhere on the page, then View Element Code).
- In the window that appears, click the phone icon.
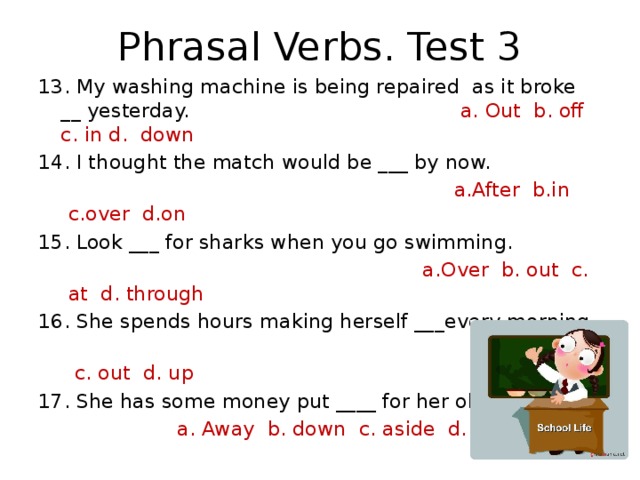
- Refresh the page. nine0008
- Start uploading photos.
How to upload a photo to Instagram* through Yandex.Browser
To start uploading a photo:
- Log in to Instagram* through a browser on your computer.
- Press F12 (or Shift+Ctrl+I, or right-click anywhere on the page, then "Inspect Element"). nine0008
- In the window that appears, click the phone icon.
- Refresh the page.
- Use.
Using Chrome extensions
If you have Chrome browser in your arsenal, you can use the extensions for posting photos on Instagram*. There are many of them, but most of them work crookedly, or do not work at all. nine0003
Consider installing and working with extensions using Upload Photo to Instagram* as an example.
After downloading, go to Extensions and turn on the download.
You can go to Extensions in Settings via Additional tools, or click on the Extensions panel icon in the form of a mosaic piece Find the desired Extension and enable it by clicking on the Button linesClicking on the extension icon will open a new browser tab with the main page of Instagram*. If you have logged in to your Instagram profile * in the browser, then it will open.
The icon for adding a new photo to the Feed will appear near the Settings on your Profile page By clicking on it, the window for adding a new post to the Feed will open right hereOf course, with this method of loading content, we are not talking about filters and some complicated settings. You can only add an image, make a caption and tag people in the photo if necessary. nine0003
Another extension is App Phone for Instagram* or [App Instagram*]. (https://chrome.google.com/webstore/detail/app-for-instagram/fhkhmblpnhfedddndenodedcaknclgkd).
Install it in the same way as above.
Add it to the Extensions panel After clicking on the extension icon, a new tab will open with the image of a smartphone. Add a photo from your computer as if you are using a smartphoneUsing the delayed posting service
The delayed posting service is designed to make it easier to manage your Instagram accounts*. You plan today, and see the result tomorrow (and even a year later). nine0003
SMMplanner allows you to add and remove photos and videos for a minimum amount, publish them to the Feed, Stories and IGTV.
Convenient and intuitive interfaceYou can apply all sorts of filters, settings to the content, edit the general appearance of the page and, of course, make many posts at once and set the time of their publication.
Read more about the service in the article "How to upload photos to Instagram* from a computer" on the SMMplanner blog.
![]()
But back to the topic of the article: how exactly to publish a photo on Instagram* through SMMplanner. nine0003
After registering with the service, in the Accounts tab, select "Connect account".
Please note that through the service you can post photos not only on Instagram * Enter the data from your profile on the social network and click "Connect" Go to the Posts tab and click "Schedule a post"You will see a window for planning a new post post. Everything is simple here and there are hints everywhere, no dancing with a tambourine.
Add photo via Photo/Video button, write text and postDespite the fact that the service is paid, you can use it for free.
The guys from SMMplanner provide two free plans, you just have to chooseWith Creator Studio
The official service from Facebook*. All work takes place in the browser, after authorization in Creator Studio, you can add and delete photos, set up delayed posting and see page statistics.
Following the instructions, enter the Login and Password for the desired Instagram account*, and confirm the link to Facebook* from your smartphone. Subsequently, you can switch between Instagram * and Facebook * through these two icons on top.
In the window that opens, add a picture using the Create Publication button, add text and publishFeatures of the service:
- you will have to authorize via Facebook*, create a page and link Instagram* and Facebook* accounts to each other; nine0008
- only works with business and Author accounts;
- Stories cannot be published;
- there are additional restrictions due to Instagram permissions*.
You can switch to the Author's account in the following way. Go to Instagram Settings* on your smartphone, select "Account" and then "Switch to Professional Account".
With the help of Instaplus.me promotion service
Instaplus is an automated online promotion service for Instagram*. It can automatically like, subscribe and unsubscribe, watch Stories, and much more.
We are interested in the ability to upload photos from a computer to Instagram* using Instaplus. On the main page, through the "Try for free" button, register, and then link your Instagram account *.
You can add a photo via Instaplus as follows:
Then you can edit the photo, add a description and a geotag.
Using the Android and iOS emulator
Using the Android application emulator would be more popular, if not for one "but", about which a little later. The essence of the emulator is as follows: by installing the program on your computer, you will work with applications on the monitor as if you were sitting on your phone. nine0003
Consider BlueStacks.
After installing the application, log in to your Google Play account In the search, enter the word "Instagram *" and install the application You can enter Instagram* by clicking on the Notifications icon ... ... section My gamesTo upload images to Instagram*, you must first add these photos to the emulator's library.
Enter Media Manager, yellow icon in My Games Click "Import from Windows" and select the photos you want You will see them in the imported files here Now go to the Instagram* emulator and upload photos the same way you would on your phone: a plus sign at the bottom in the center of the screen , and the Gallery will already have the necessary photosThere is another way. When you launch Instagram* through the emulator, and press the usual plus sign at the bottom of the screen to create a new post, open the Gallery.
Select "Others" and expand the list as shown in screenshot Click Select from Windows, and mark the desired photos on your computer Now they are stored in the SharedFolder section You can find this section in the list from the Gallery when creating a post.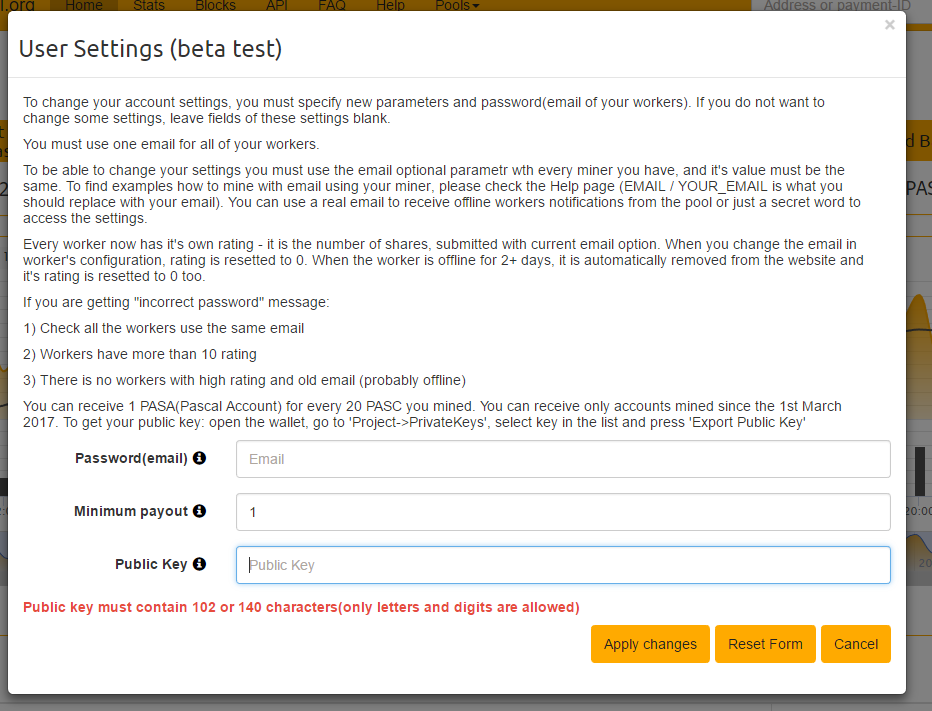
Now that "but" - the moment about the performance of your computer. If you have 4 GB. RAM and less, then each process in the emulator will take you 5 minutes or more; in other words, everything will slow down, and the program may refuse to work at all. nine0003
With the help of online services
Here the situation is the same as with browser extensions - there are many online services, but few of them work. Consider the principle on the example of instap.ru.
The service was created specifically for working with Instagram accounts*. Please register before using the service for the first time.
To add a photo from your computer, enter your Instagram Login and Password* in the required window and click "Add". Next "Download from PC" After selecting the desired image, you will be able to access the settings window Through the service, you can add a description, mark users, publish photos both in the Feed and in Stories. But such free services have bugs. This will upset that after applying filters to a photo, it is published without them.
Disadvantages of the service: no more than three posts per day without a premium plan, you can't create a photo gallery and apply settings from Stories to photos when posting there.
Using applications from the Microsoft website
Until recently, by installing the official Instagram* application from Microsoft, you could upload photos and videos from your computer to Instagram*. The creators have updated it, and now there is no such possibility left. Despite the outrage in the product reviews, no one is going to return the ability to upload photos. But this method cannot be discounted yet. As they say, what if? nine0003
It's worth noting that you can also download other apps from the Microsoft Store that allow you to upload photos to Instagram* from your computer.
App for Instagram*: View, Upload Photo, which should allow you to upload images. By the way, this is the only function of this application, in addition to viewing the Feed and Profile.
Winsta is an unofficial Instagram client* for Windows 10.
The application is paid, but you can use the free period of 7 daysIn the application, you can add an image, edit its size and make a description for the post.
Winsta has only an English-language interface, adding a new photo via the Feed buttonLet's summarize
There are many ways to upload photos to Instagram* from a computer, for clarity, we will collect them in a single table and compare the possibilities of the methods with the functionality of the Instagram* application on a smartphone.
|
It should be noted that the data in the table indicate only the possibility of a particular action, and does not mean the convenience of using the service or application. nine0003
For example, using the emulator, you will be “like in a smartphone”, but firstly, it may simply not work for you, and secondly, it has only the standard functionality of the application, while SMMplanner, Creator Studio or Instaplus can offer opportunities that go beyond the standard.
And if you need to upload a photo once, then there is no need for great functionality: the ability to upload through a browser is enough.
nine0000 Why Instagram crops photos - Let's figure it out
Content
- 1 How to post a photo on Instagram in full size
- 2 How to post a photo on Instagram without ruining its quality
- 3 How to post a photo in full growth (vertical) on Instagram
- 4 How to upload a story photo without cropping it
Instagram crops the photo? This is due to the fact that the maximum size of a photo that can be fully uploaded to the Instagram social network is 1080 by 1080. Moreover, such a download is possible if it is carried out using a mobile phone or tablet. The user will not be able to upload photos from a laptop. Therefore, in order to fully upload a photo without losing its quality and original size, it must meet these parameters. nine0003
If the photo has a lower or higher resolution, the photo is automatically cropped on Instagram and it will not load correctly. Such a photo will have the shape of a square. This is necessary so that users, accessing the social network from different devices, can comfortably view photos.
In addition, Instagram imposes requirements not only on the resolution of photographs, but also on their format and editing method. On a mobile phone, all photos are saved in JPEG format. Such photos, when repeatedly edited and further saved, change their quality in the direction of deterioration. After all, when a person saves a photograph, it is archived again. From this we can conclude that it is better to edit and upload photos from a popular social network right away. This can be done using a user-friendly interface and an editor that can be used immediately after downloading. nine0003
Content
- How to post a photo on Instagram in full size
- How to post a photo on Instagram without ruining its quality
- How to post a photo in full height (vertical) on Instagram
- How to upload a photo story without cropping it
How to post a photo on Instagram in full size
Almost all users of the popular photo network faced a situation when they posted a seemingly perfect photo, and the service distorted it beyond recognition or changed its size. As mentioned above, Instagram crops a photo if its frame does not match the resolution of 1080 by 1080. Modern gadgets take pictures with a resolution of 1100 or even higher. The extra edge is cut off by the service and as a result a distorted photograph is obtained. How to make sure that Instagram does not crop the photo when you post it? nine0003
There is a solution! In order to post a photo in full size, there are special applications:
- NoCrop is a free program with additional paid features. After downloading and installing it, a person needs to select a photo that he wants to upload to Instagram. A white frame appears around the photo, the size of which can be adjusted independently. In any case, no significant part will remain behind the scenes. Next, you just need to save the photo and upload it to the social network
.
- InstaSize - the application also works for free. It is available for gadgets with Android operating system and iQS.
In addition to the usual functions, the user can also use additional ones: change the color and shape of the borders, as well as their size, add an inscription, and so on.
- Whitegram - also works for free. Such an application will appeal to users who love minimalism. It has no extra features. The program is intended only for fitting photographs to the requirements of Insta. However, it retains the quality of the photos well when uploading. nine0008
How to post a photo on Instagram without ruining its quality
In addition to the fact that the social network changes their resolution when uploading photos, it can significantly degrade their quality. This is primarily due to technical failures on the service. After all, in order not to waste time, the program compresses the file and also quickly downloads it. The number of pixels is reduced. Therefore, the quality becomes different.
The file format also matters if it is loaded correctly. For Insta, the PNG format is preferred, not JPEG, like many mobile devices. nine0003
What is the best way to keep the quality of uploaded photos? For gadgets with the Android operating system, you can customize the download so that the photos are no longer cloudy and deformed. This is done as follows:
- Open the Instagram program,
- In the settings, find the "Advanced settings" tab,
- From the two proposed options, select the inscription "Using high-quality image processing",
- Upload the desired photo to the social network. nine0008
Why doesn't Instagram upload full videos?
Newbies who just signed up for the photo network cannot understand why Instagram cuts the video. The thing is that the length of the video on the social network is strictly limited. The user has the right to upload a video to his profile, the duration of which does not exceed one minute. You can download the rest with the following file. Thus, while watching a video, subscribers will be able to watch its continuation by simply flipping the page with the video. nine0003
How to post a full-length (vertical) photo on Insta
Most recently, employees of the social network Instagram noticed that users began to often cancel the upload of photos if they saw that the photo would not look like it originally looked. Previously, when uploading a vertical photo, it was cut off and Instagram made it square. Let's say a girl wanted to post a full-length photo, and the service cut off her legs or, worse, half her head. But this problem was solved thanks to the constant monitoring of user behavior. nine0003
An updated version of the Insta application is now available, in which a person can choose the proportions of photos. All you need to do is, after uploading a photo, click on the icon of a square with two arrows. This icon is located in the lower left corner of the download interface. After clicking on it, you should select the preferred aspect ratio of the file and confirm the download. The same applies to videos.
How to upload a photo story without cropping it
Instagram also has certain requirements for photo stories.
![]()